{
"cells": [
{
"cell_type": "markdown",
"metadata": {},
"source": [
"# Lecture 3\n",
""
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"-Uploading files to CoCalc: [>>](#Uploading-files-to-CoCalc) \n",
"-Including images: [>>](#Including-images) \n",
"-Code and markdown cells: [>>](#Code-and-markdown-cells) \n",
"-Lists: [>>](#Lists) \n",
"-Tuples: [>>](#Tuples) \n",
"-NumPy arrays: [>>](#NumPy-arrays) "
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Uploading files to CoCalc\n",
"\n",
"Use the *upload* button!"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Including images\n",
"\n",
"The simplest way is to use markdown.\n",
"\n",
"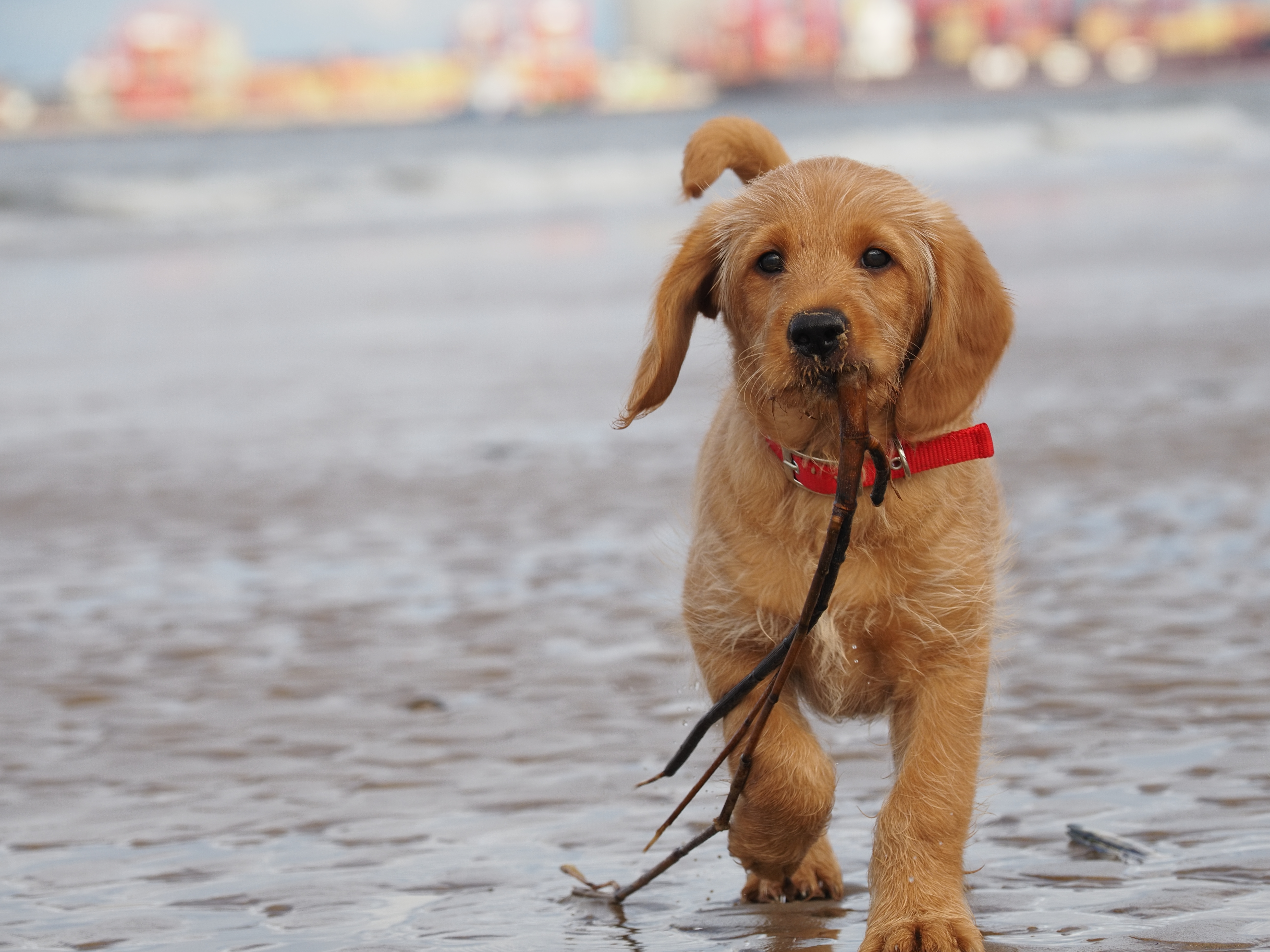"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"If you need to change the size, use an *html* image (`img`).\n",
"\n",
"
\n",
"\n",
"If you want to do something a bit more exotic, use an *html* `figure`.\n",
"\n",
"
\n",
"
\n",
"Figure 1: Jasper with seaweed (left) and superman (right).\n",
" "
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Code and markdown cells\n",
"\n",
"Switch between these using *cell* menu commands or the shortcuts:\n",
"\n",
"* Convert to markown, *Esc*, *m*, *Enter*.\n",
"* Convert to code, *Esc*, *y*. "
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Lists\n",
"\n",
"Create a list:"
]
},
{
"cell_type": "code",
"execution_count": 1,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"this_list = [3, 1.0, 'green', 'eggs']\n"
]
}
],
"source": [
"this_list = [3, 1.0, 'green', \"eggs\"]\n",
"print(\" \")\n",
"print(\"this_list =\",this_list)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Access list elements"
]
},
{
"cell_type": "code",
"execution_count": 2,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"this_list[2] = green\n"
]
}
],
"source": [
"print(\" \")\n",
"print(\"this_list[2] =\",this_list[2])"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Add list elements:"
]
},
{
"cell_type": "code",
"execution_count": 3,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"this_list[0] + this_list[1] = 4.0\n"
]
}
],
"source": [
"print(\" \")\n",
"print(\"this_list[0] + this_list[1] =\",this_list[0] + this_list[1])"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"But what \"+\" means depends on the type of the elements!"
]
},
{
"cell_type": "code",
"execution_count": 4,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"this_list[2] + this_list[3] = greeneggs\n"
]
}
],
"source": [
"print(\" \")\n",
"print(\"this_list[2] + this_list[3] =\",this_list[2] + this_list[3])"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Need to put in space (\" \" or ' ') explicitly!"
]
},
{
"cell_type": "code",
"execution_count": 5,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"this_list[2] + ' ' + this_list[3] = green eggs\n"
]
}
],
"source": [
"print(\" \")\n",
"print(\"this_list[2] + ' ' + this_list[3] =\",this_list[2] + ' ' + this_list[3])"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"And \"+\" won't work if it doesn't make sense!"
]
},
{
"cell_type": "code",
"execution_count": 6,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n"
]
},
{
"ename": "TypeError",
"evalue": "unsupported operand type(s) for +: 'float' and 'str'",
"output_type": "error",
"traceback": [
"\u001b[1;31m---------------------------------------------------------------------------\u001b[0m",
"\u001b[1;31mTypeError\u001b[0m Traceback (most recent call last)",
"\u001b[1;32m\u001b[0m in \u001b[0;36m\u001b[1;34m\u001b[0m\n\u001b[0;32m 1\u001b[0m \u001b[0mprint\u001b[0m\u001b[1;33m(\u001b[0m\u001b[1;34m\" \"\u001b[0m\u001b[1;33m)\u001b[0m\u001b[1;33m\u001b[0m\u001b[1;33m\u001b[0m\u001b[0m\n\u001b[1;32m----> 2\u001b[1;33m \u001b[0mprint\u001b[0m\u001b[1;33m(\u001b[0m\u001b[1;34m\"this_list[1] + this_list[2] =\"\u001b[0m\u001b[1;33m,\u001b[0m\u001b[0mthis_list\u001b[0m\u001b[1;33m[\u001b[0m\u001b[1;36m1\u001b[0m\u001b[1;33m]\u001b[0m \u001b[1;33m+\u001b[0m \u001b[0mthis_list\u001b[0m\u001b[1;33m[\u001b[0m\u001b[1;36m2\u001b[0m\u001b[1;33m]\u001b[0m\u001b[1;33m)\u001b[0m\u001b[1;33m\u001b[0m\u001b[1;33m\u001b[0m\u001b[0m\n\u001b[0m",
"\u001b[1;31mTypeError\u001b[0m: unsupported operand type(s) for +: 'float' and 'str'"
]
}
],
"source": [
"print(\" \")\n",
"print(\"this_list[1] + this_list[2] =\",this_list[1] + this_list[2])"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Change an element:"
]
},
{
"cell_type": "code",
"execution_count": 7,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"this_list[2] + ' ' + this_list[3] = cream eggs\n"
]
}
],
"source": [
"this_list[2] = \"cream\"\n",
"print(\" \")\n",
"print(\"this_list[2] + ' ' + this_list[3] =\",this_list[2] + ' ' + this_list[3])"
]
},
{
"cell_type": "code",
"execution_count": 8,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"this_list[2] + this_list[3] = cream eggs\n"
]
}
],
"source": [
"this_list[2] = \"cream \"\n",
"print(\" \")\n",
"print(\"this_list[2] + this_list[3] =\",this_list[2] + this_list[3])"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Tuples\n",
"\n",
"Create a tuple:"
]
},
{
"cell_type": "code",
"execution_count": 9,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"The contents of this tuple are (3, 1, 'green', 'eggs')\n"
]
}
],
"source": [
"this_tuple = (3, 1, \"green\", \"eggs\")\n",
"print(\" \")\n",
"print(\"The contents of this tuple are\", this_tuple)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Access tuple elements:"
]
},
{
"cell_type": "code",
"execution_count": 10,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"this_tuple[2] = green\n"
]
}
],
"source": [
"print(\" \")\n",
"print(\"this_tuple[2] =\",this_tuple[2])"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Add tuple elements:"
]
},
{
"cell_type": "code",
"execution_count": 11,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"this_tuple[0] + this_tuple[1] = 4\n"
]
}
],
"source": [
"print(\" \")\n",
"print(\"this_tuple[0] + this_tuple[1] =\",this_tuple[0] + this_tuple[1])"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Cannot change the contents of a tuple!"
]
},
{
"cell_type": "code",
"execution_count": 12,
"metadata": {},
"outputs": [
{
"ename": "TypeError",
"evalue": "'tuple' object does not support item assignment",
"output_type": "error",
"traceback": [
"\u001b[1;31m---------------------------------------------------------------------------\u001b[0m",
"\u001b[1;31mTypeError\u001b[0m Traceback (most recent call last)",
"\u001b[1;32m\u001b[0m in \u001b[0;36m\u001b[1;34m\u001b[0m\n\u001b[1;32m----> 1\u001b[1;33m \u001b[0mthis_tuple\u001b[0m\u001b[1;33m[\u001b[0m\u001b[1;36m2\u001b[0m\u001b[1;33m]\u001b[0m \u001b[1;33m=\u001b[0m \u001b[1;34m\"cream\"\u001b[0m\u001b[1;33m\u001b[0m\u001b[1;33m\u001b[0m\u001b[0m\n\u001b[0m\u001b[0;32m 2\u001b[0m \u001b[0mprint\u001b[0m\u001b[1;33m(\u001b[0m\u001b[1;34m\" \"\u001b[0m\u001b[1;33m)\u001b[0m\u001b[1;33m\u001b[0m\u001b[1;33m\u001b[0m\u001b[0m\n\u001b[0;32m 3\u001b[0m \u001b[0mprint\u001b[0m\u001b[1;33m(\u001b[0m\u001b[1;34m\"this_tuple[2] + ' ' + this_tuple[3] =\"\u001b[0m\u001b[1;33m,\u001b[0m\u001b[0mthis_tuple\u001b[0m\u001b[1;33m[\u001b[0m\u001b[1;36m2\u001b[0m\u001b[1;33m]\u001b[0m \u001b[1;33m+\u001b[0m \u001b[1;34m' '\u001b[0m \u001b[1;33m+\u001b[0m \u001b[0mthis_tuple\u001b[0m\u001b[1;33m[\u001b[0m\u001b[1;36m3\u001b[0m\u001b[1;33m]\u001b[0m\u001b[1;33m)\u001b[0m\u001b[1;33m\u001b[0m\u001b[1;33m\u001b[0m\u001b[0m\n",
"\u001b[1;31mTypeError\u001b[0m: 'tuple' object does not support item assignment"
]
}
],
"source": [
"this_tuple[2] = \"cream\"\n",
"print(\" \")\n",
"print(\"this_tuple[2] + ' ' + this_tuple[3] =\",this_tuple[2] + ' ' + this_tuple[3])"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## NumPy arrays\n",
"\n",
"Import `numpy`!"
]
},
{
"cell_type": "code",
"execution_count": 13,
"metadata": {},
"outputs": [],
"source": [
"import numpy as np"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Create array from list:"
]
},
{
"cell_type": "code",
"execution_count": 14,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"This numpy array is [ 1. 1.2 -4.1]\n"
]
}
],
"source": [
"array_list = np.array([1.0, 1.2, -4.1])\n",
"print(\" \")\n",
"print(\"This numpy array is\",array_list)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Access array elements:"
]
},
{
"cell_type": "code",
"execution_count": 15,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"array_list[0] = 1.0\n",
" \n",
"array_list[1] = 1.2\n",
" \n",
"array_list[1] + array_list[2] = -2.8999999999999995\n"
]
}
],
"source": [
"print(\" \")\n",
"print(\"array_list[0] =\",array_list[0])\n",
"print(\" \")\n",
"print(\"array_list[1] =\",array_list[1])\n",
"print(\" \")\n",
"print(\"array_list[1] + array_list[2] =\",array_list[1] + array_list[2])"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"\"Add\" elements:"
]
},
{
"cell_type": "code",
"execution_count": 16,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"array_new_list = ['1.0' 'cheese' '-17.0']\n",
" \n",
"array_new_list[1] + array_new_list[2] = cheese-17.0\n"
]
}
],
"source": [
"array_new_list = np.array([1.0, \"cheese\", -17.0])\n",
"print(\" \")\n",
"print(\"array_new_list =\",array_new_list)\n",
"print(\" \")\n",
"print(\"array_new_list[1] + array_new_list[2] =\",array_new_list[1] + array_new_list[2])"
]
},
{
"cell_type": "code",
"execution_count": 17,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
" \n",
"array_list[1] = 7.0\n"
]
}
],
"source": [
"x = 7\n",
"array_list[1] = x\n",
"print(\" \")\n",
"print(\"array_list[1] =\",array_list[1])"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [],
"source": []
}
],
"metadata": {
"anaconda-cloud": {},
"kernelspec": {
"display_name": "Python 3",
"language": "python",
"name": "python3"
},
"language_info": {
"codemirror_mode": {
"name": "ipython",
"version": 3
},
"file_extension": ".py",
"mimetype": "text/x-python",
"name": "python",
"nbconvert_exporter": "python",
"pygments_lexer": "ipython3",
"version": "3.8.5"
}
},
"nbformat": 4,
"nbformat_minor": 4
}